COMP 110-003 Spring 2013
Program 2: GUI Calculator
75 points
Assigned: Tuesday, January 29
Due: Sunday, February 17
Description
Write a GUI-based program that mimics a calculator. The program should
take as input two floating-point numbers and the operation to be performed.
It should then output the numbers, the operator, and the result (formatted
to two decimal places).
The user input should be in the format operand1 operator operand2,
where the operands and the operator are separated by spaces. For example,
to add 2 and 3, the user should enter 2 + 3 in the text
field of the dialog box.
You should support the following operations: addition (+), subtraction
(-), multiplication (*), division (/), and mod (%).
Special cases to handle:
For division, if the denominator is zero, print an appropriate message.
If the user enters an invalid operator (i.e., anything not +, -,
*, /, %), print an appropriate message.
Since the user will enter floating-point numbers, you will have to convert the numbers to integers when performing modular arithmetic.
You are not responsible for making sure that the user enters numbers, instead
of letters, for the operands. This would cause a "NumberFormatException"
when trying to tokenize the input string.
Here's an example run:
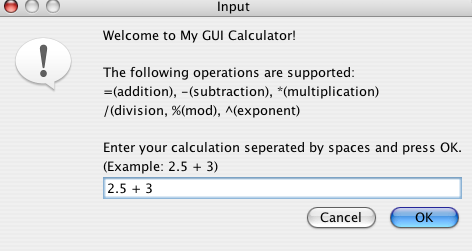
Extra Credit:
Add the ^ operator to your code. There are Java packages that include the ^ operator (Math.pow()), however you cannot use them for this extra credit. You will get the credit only if you use loop statements. When testing the ^ operator use small numbers!
Note: operand1 ^ operand2 means that
operand1
should be raised to the operand2(th) power. Assume that operand2 is an integer.
What to Turn in
-
Your yourlastname_program2.jar file that includes your Calculator.java source file
- A text file named yourlastname_program2.txt that has a short write-up of questions you had and/or problems you encountered while doing this assignment. Create it from eclipse, and include it in the jar file.
What to Do
- Name your Java source file Calculator.java and your class Calculator.
- Submit via Sakai your jar file by February 17, 2013 11:59pm
- Your jar file should include Calculator.java, Calculator.class, MANIFEST.MF, and yourlastname_program2.txt
- As always, use these instructions to create your jar file.
Requirements
When I run/examine your program, it must satisfy the following requirements.
The maximum point value for each is shown in brackets.
-
[5] Your class, Java source file, and Jar file must be appropriately named
(as specified above).
-
[10] You must use the JOptionPane class to create dialog boxes
for user input and output. (See Lab 2) Your user input dialog box should contain instructions
for using the program, including a list of the supported operations.
- [10] You must correctly parse the user input String.
-
[10] You must display the correct result of the calculation to the user.
-
[5] If the user attempts to divide by 0, you must print a message to
that effect (e.g., "division by 0 not allowed").
-
[10] If the user enters an invalid operator, you must print a message
to that effect (e.g., "operation not supported").
-
[10] You must format the result of the calculation to two decimal places.
-
[5] You must convert floating-point numbers to integers to perform modular arithmetic.
-
[5] You must use meaningful variable names, which conform to the style
guidelines and Java naming convention discussed in class.
-
[5] You must comment your code, including block-like multi-line comments
and single-line comments where appropriate. In addition, your code must
be neatly and clearly formatted using appropriate "white space."
-
[5] Extra Credit.
Notes:
-
If your program doesn't compile or run (i.e., has a syntax error),
you will receive 0 credit for the items listed above in bold (so, at most
15/75 points).
-
Remember that an implicit requirement for all assignments is that your
code must include (at the top) the standard
program header with pledge